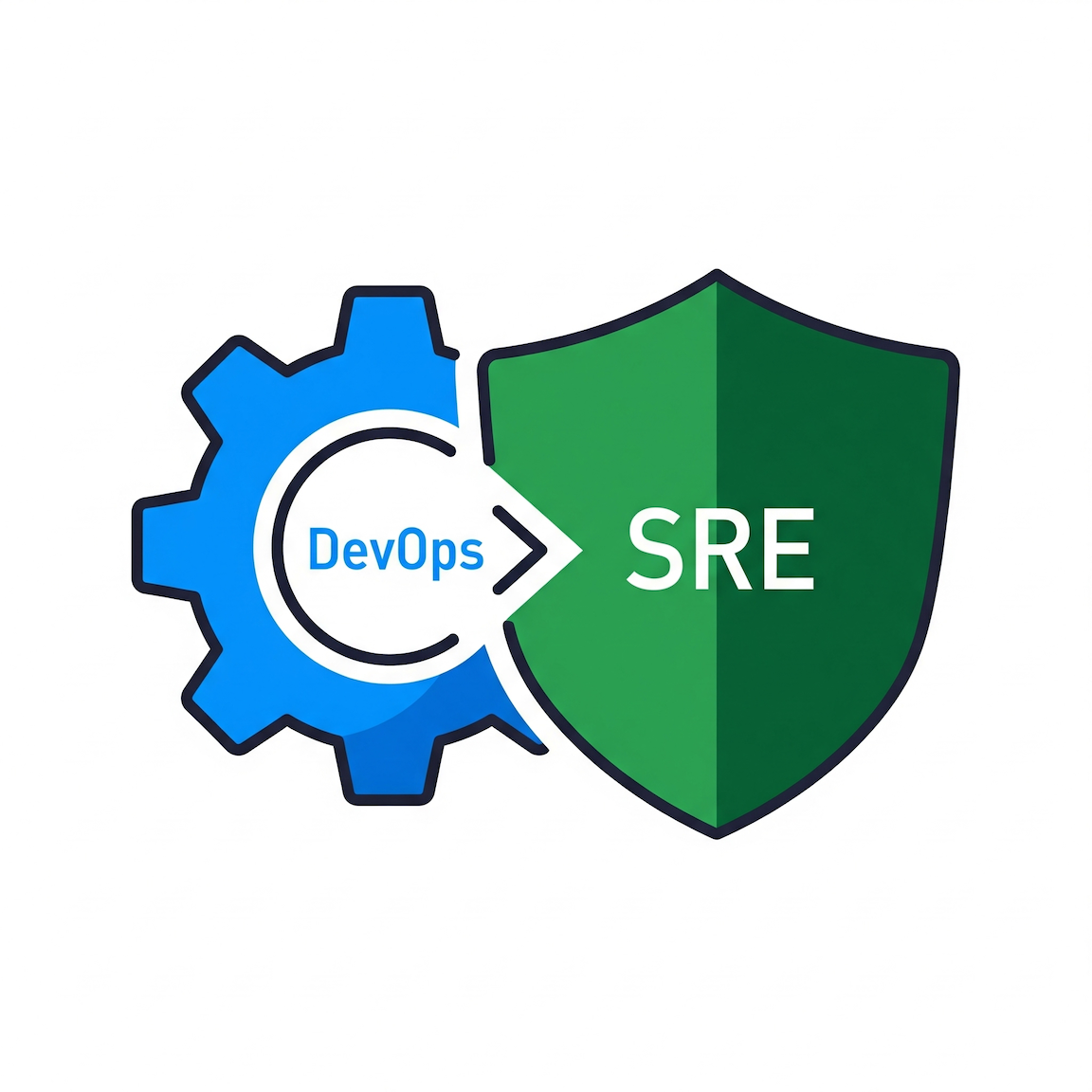
Terminal-Based Development with Neovim, tmux, and CLI Tools
March 23, 2025
My development workflow is centered around terminal-based tools that maximize productivity and minimize context-switching. This post details my setup and how it enhances my software engineering workflow.
Why Terminal-Based Development?
GUI applications and IDEs offer convenience, but a terminal-centric approach provides several distinct advantages:
- Efficiency: Keyboard-driven workflows eliminate mouse dependencies and reduce context switching
- Resource Efficiency: Terminal applications use minimal system resources
- Customizability: Complete control over every aspect of the development environment
- Transferability: Skills and configurations work across different machines and operating systems
- Remote Work: Seamless experience when working on remote servers via SSH
Core Components
Neovim as IDE
Neovim serves as my primary text editor and IDE. The key advantages include:
- Modal editing for efficient text manipulation
- Plugin ecosystem that provides modern IDE features
- Highly customizable with Lua configurations
- Minimal resource usage compared to GUI editors
My essential Neovim plugins include:
-- Plugin management
use 'wbthomason/packer.nvim'
-- LSP Configuration
use 'neovim/nvim-lspconfig'
use 'hrsh7th/nvim-cmp'
-- File navigation
use {
'nvim-telescope/telescope.nvim',
requires = { 'nvim-lua/plenary.nvim' }
}
use 'ThePrimeagen/harpoon'
-- Syntax highlighting
use {
'nvim-treesitter/nvim-treesitter',
run = ':TSUpdate'
}
-- Git integration
use 'lewis6991/gitsigns.nvim'
use 'tpope/vim-fugitive'
tmux for Session Management
tmux allows me to maintain multiple terminal sessions and window layouts:
- Persistent sessions that survive disconnections
- Custom layouts for different development tasks
- Split panes for simultaneous terminal access
- Session templates for quick project setup
My .tmux.conf
includes these essential configurations:
# Change prefix to Ctrl+a
unbind C-b
set -g prefix C-a
bind C-a send-prefix
# Mouse mode
set -g mouse on
# Split panes using | and -
bind | split-window -h
bind - split-window -v
unbind '"'
unbind %
# Vim-style pane navigation
bind h select-pane -L
bind j select-pane -D
bind k select-pane -U
bind l select-pane -R
Command-Line Tools
Essential CLI tools that enhance my workflow:
- ripgrep: Fast code searching with intelligent defaults
- fd: Intuitive alternative to
find
- fzf: Fuzzy finder for files, history, and more
- lazygit: Terminal UI for Git operations
- HTTPie: User-friendly command-line HTTP client
Benefits for Software Engineering
This terminal-centric approach provides several advantages for software engineering tasks:
- Speed and Efficiency: Keyboard shortcuts and command-line operations reduce time spent on navigation and manipulation
- Focused Environment: Minimal UI reduces distractions
- Reproducibility: Configurations can be version-controlled and shared
- Scriptability: Every action can be automated with shell scripts
- Resource Optimization: Low resource usage leaves more computing power for development tasks
Sample Development Workflow
Here’s how I might work on a Ruby project:
- Start a named tmux session with a custom layout:
tmux new-session -s ruby-project -n editor
- Set up window panes for:
- Code editing (Neovim)
- Test runner (continuous testing)
- Git operations (lazygit)
- Command execution (IRB/Rails console)
- Use Neovim with:
- LSP for code intelligence
- Telescope for file navigation
- Treesitter for syntax highlighting
- Custom keybindings for Ruby-specific operations
-
Run tests and see results without leaving the terminal environment
- Commit changes using lazygit or git commands directly
Getting Started with Terminal-Based Development
If you’re interested in trying this approach, start with these steps:
- Install and configure Neovim with basic plugins
- Learn tmux fundamentals for session and window management
- Gradually incorporate CLI tools as alternatives to GUI applications
- Version control your configurations with a dotfiles repository
- Continuously refine your workflow based on pain points
In future posts, I’ll dive deeper into specific aspects of this setup, including Neovim configuration for Ruby development, tmux session templates, and automation scripts for common workflows.
What’s your development environment like? Have you tried a terminal-based approach? Share your thoughts and questions in the comments!
Comments